Posted By: Joozey
How to: tiles in 3dgs - 07/25/11 17:38
Hey,
Though I just as well place it here. I'm running against problems with 3dgs when trying to show 80*60 little bitmap tiles on the screen. I want to make a little game like diggers/minecraft/dwarffortress in 2D; dig blocks and search for treasures. So I made a tileset with 8x8 tiles, and using some noise functions to create a 60*80 tiles geological landscape.
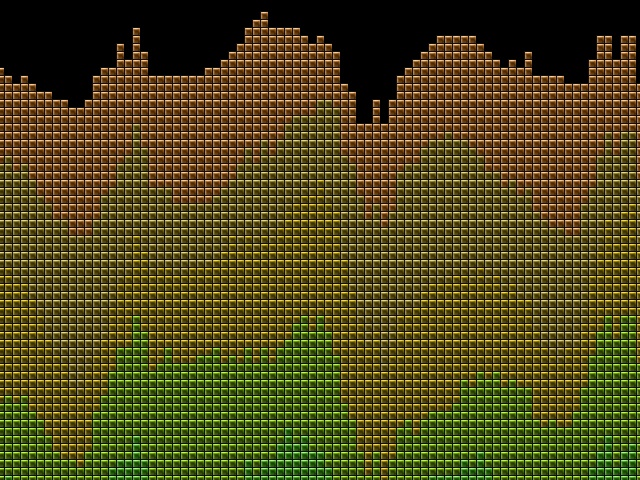
I first tried to use panels for each tile, but that soon proved to be very fps-inefficient. Then I used one panel and 80*60 windows on it. That worked significantly better, but 14 fps is not all that great. Using BMP instead of TGA saved me another 3 fps, but what else could I do to improve the framerate to at least 30? Should I approach this way differently? Any ideas?
Thanks,
Joozey
Though I just as well place it here. I'm running against problems with 3dgs when trying to show 80*60 little bitmap tiles on the screen. I want to make a little game like diggers/minecraft/dwarffortress in 2D; dig blocks and search for treasures. So I made a tileset with 8x8 tiles, and using some noise functions to create a 60*80 tiles geological landscape.
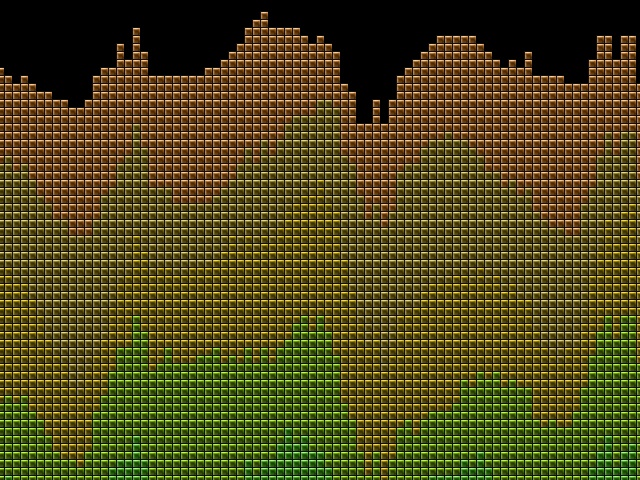
I first tried to use panels for each tile, but that soon proved to be very fps-inefficient. Then I used one panel and 80*60 windows on it. That worked significantly better, but 14 fps is not all that great. Using BMP instead of TGA saved me another 3 fps, but what else could I do to improve the framerate to at least 30? Should I approach this way differently? Any ideas?
Thanks,
Joozey